Yet for less experienced developers unfamiliar current JavaScript ecosystems, learning React often proves overwhelming initially given its steep learning curve.
This guide aims serving an easy-to-understand React JS tutorial tailored for beginners approaching the wildly popular library for the first time through the following key learning objectives:
⬇️ React’s Declarative Nature Explained
⬇️ Core Concepts Covered to Avoid Confusion
⬇️ Practical Sample App Bringing Concepts Together
Let’s get started mastering React JS!
1: React JS Explained for Beginners
At the highest level, React represents a JavaScript framework for building better component-driven user interfaces in web and mobile apps over slow clunky vanilla HTML and CSS. Technically React classified as a library given its focused scope.
In traditional web development, making even minor visual changes means manipulating HTML/CSS/JavaScript requiring full-page reloads losing all app state. React introduced the concept of a Virtual DOM – a JavaScript representation of the actual DOM. Code updates the Virtual DOM in-memory without changing the real DOM until final.
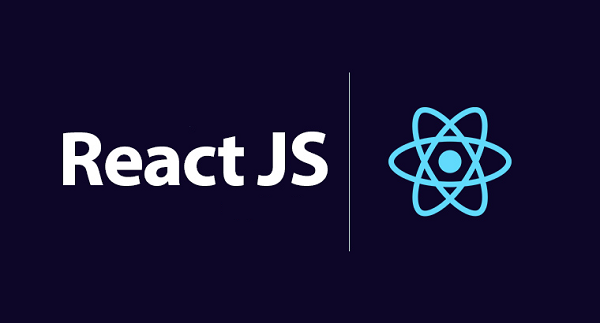
This abstraction means efficiently re-rendering only changed components instead of entire pages accelerating large web apps dramatically.
Additional beginner-friendly capabilities includes:
⚛️ Reusable UI Components keeping code DRY
⚛️ One-way Data Binding for faster state changes
⚛️ Lightweight library size at just 120kB
By leveraging React for UIs rather than raw JavaScript, sites gain improved performance scalability and code maintainability. The component model also enables excellent reusability.
Now that we covered what React does at a higher level, let’s unpack Key terminologies any beginners should understand before diving hands-on…
2: Core React Concepts and Components
Learning React involves digesting many initially foreign concepts that set its design paradigm apart from traditional web development stacks.
Grasping these core ideas right away eliminates much frustration down the road by cementing holistic understanding versus struggling through coding examples using unfamiliar patterns blindly.
While React does present a learning curve upfront, its declarative nature pays off through cleaner reusable interfaces.
Here are beginner-friendly explanations of key React terms to cement:
🔹 Components
At a basic level, you can consider React Components as equivalent to custom HTML elements helping break complex UIs into independent reusable pieces of code performing specific functions.
Components let developers cleanly componentize (+10 points for overuse) UIs into self-contained units handling a particular task rather than giant monolithic stacks hard to iterate upon.
🔹 JSX
While React leverages basic JavaScript under the hood, JSX represents an extension adding XML syntax to JavaScript for structuring component layouts and user interfaces declaratively.
This declarative structure reads similarly to HTML inside JavaScript code for templating React component UI details in a simple visual way.
🔹 Props
The key method for customizing React component functionality and design gets passed through as props allowing parent components to directly inject data and change behavior of child subcomponents.
You can consider props as function arguments for React components or configuration settings adjusting output.
🔹 State
Whereas props pass static data down nominatively to child components, a component’s state represents internal mutable data that changes over time initiating re-renders upon updates.
Stateful components equip reactive UIs that re-compute display when changed internally vs externally through new props values.
3: Hands-On React Learning with Example To-Do App
While glossing over concepts theoretically offers a starting point digesting React for total beginners, tangible coding application through a starter app example brings all the core ideas together practically solidifying understanding.
Let’s explore a simplified To-Do web app leveraging key facets of React including components, props, state, JSX and more by building the basics step-by-step:
Setup
We start by importing React libraries and dependencies either via CDNs for quick prototyping or through module bundlers for more productionized environments:
import React from 'react';
import ReactDOM from 'react-dom';
The bare minimum markup to launch a React app requires a root DOM node to inject everything into:
<div id="root"></div>
We can trigger this render from our JS code:
ReactDOM.render(<h1>Hello React!</h1>, document.getElementById('root'));
This will inject the h1 element into the root div.
Components
As highlighted earlier, components facilitate UI decomposition into reusable self-contained units. We can create a simple Title component in JSX:
function Title() {
return (
<h1>My First React App</h1>
);
}
And compose this component within root render call:
ReactDOM.render(
<Title />,
document.getElementById('root')
);
This component breakdown makes complex UIs much more manageable!
Props
To demonstrate how data gets passed into components, we can create a custom Greeting component expecting a name prop:
function Greeting(props) {
return <h1>Hi there, {props.name}!</h1>;
}
Then passed from parent:
<Greeting name="Sarah" />
Rendering personalized welcome text!
State
To manage changing data, our components can define state hooks:
import { useState } from 'react';
function Form() {
const [name, setName] = useState('Mary');
return (
<input
value={name}
onChange={e => setName(e.target.value)} />
);
}
Here any updates to the input change component state kicking off efficient re-rendering.
Hopefully walking through sample React code demystifies many foundational concepts and shows cleaner declarative structures possible for building web UIs vs traditional imperative JavaScript!
4: Level Up React JS Skills with Next Steps
This intro guide aimed an easily digestible React JS overview for complete beginners navigating key paradigm shifts needed when leveraging React for modern web development.
We unpacked what React does, clarified potentially confusing jargon with beginner examples and brought ideas together practically by demonstrating components, state and props collaborating in a basic To-Do app walkthrough.
Of course an enormous depth exists learning React beyond basics that this tutorial introduced. I highly suggest exploring additional resources for leveling up React mastery:
🔹 Start small personal projects experimenting reactively
🔹 Explore hooks for advanced stateful logic
🔹 Tackle styling solutions like CSS Modules
🔹 Learn ES6 syntax standards deeply
🔹 Integrate React Router for multi-page apps
🔹 Look into Flux/Redux for app data flows
🔹 Understand create-react-app bootstrapping
🔹 Debug effectively with React DevTools
The documentation and community around React also offer guides taking coding skills to the next level.
With this beginner-optimized jumping off point now covered walking through React fundamentals, what related topics would you like explained for mastering professional-grade implementations? Share what unsure areas need clarification in the comments!